ショートカット(.lnk)ファイルの情報を取得して、情報を使いたい場面があります。今回は、.lnkファイルの情報を取得する方法を残しておきます。
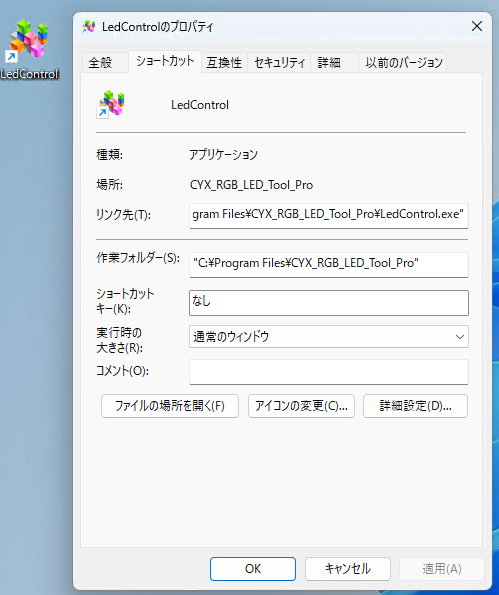
内容
ショートカット(.lnk)ファイルのプロパティ情報を使って、外部プログラムからショートカットのプログラムを起動させるような機能を作りたい場合があります。(特にランチャーのような場合)
プログラムのアイコンを取得する方法が、意外とわかりずらかったのでメモしておきます。
IWshRuntimeLibraryを参照する
ショートカットのプロパティを取得するためにIWshRuntimeLibraryへの参照が必要になります。
手順を記述します。
「ソリューションエクスプローラ」の「参照」を右クリックします。
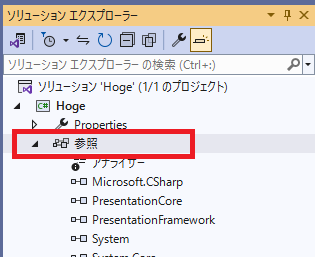
「参照の追加」を選択します。
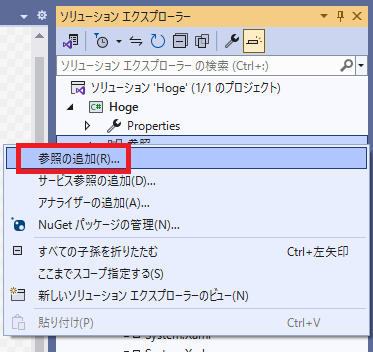
「参照マネージャ」ウィンドウが開きますので、「COM」を選択しリストビューの中から”Windows Script Host Object Model”にチェックを入れて、[OK]をクリックします。
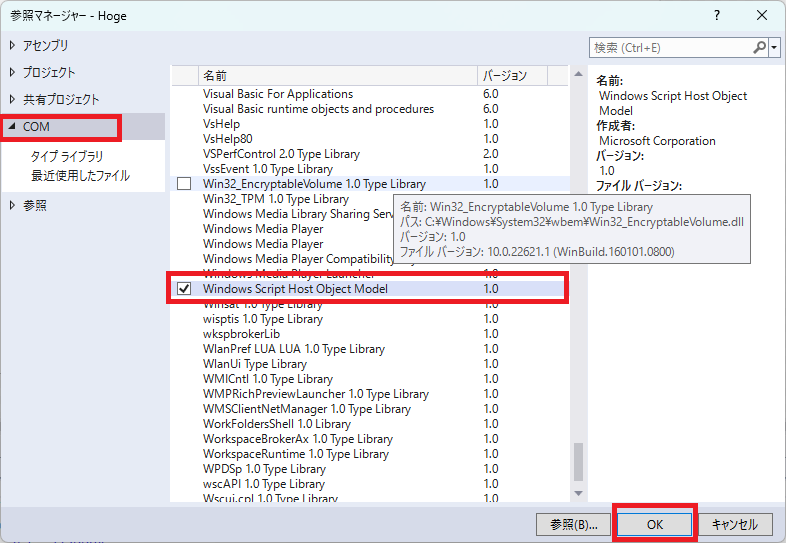
「ソリューションエクスプローラー」の「参照」に”IWshRuntimeLibrary“が追加されます。
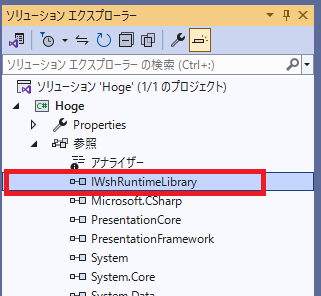
これで、ショートカットのプロパティを取得する準備が完了しました。
プログラム
using System.IO;
using System.Windows.Media.Imaging;
using System.Drawing;
using System.Windows.Interop;
public class ShortCutData
{
public String Name { get; set; }
public String File { get; set; }
public String Path { get; set; }
public BitmapSource Img { get; set; }
}
public static ShortCutData GetShortCut(String path)
{
ShortCutData sc = new ShortCutData();
sc.Name = Path.GetFileNameWithoutExtension(path);
sc.FileName = Path.GetFileName(path);
WshShell ws = new IWshRuntimeLibrary.WshShell();
IWshShortcut wsc = (IWshShortcut)ws.CreateShortcut(path);
// リンクの取得
String GetIconPath = "";
sc.Path = String.Format("{0}", wsc.TargetPath);
GetIconPath = sc.Path;
String ex = Path.GetExtension(sc.Path);
if(String.IsNullOrEmpty(Path.GetExtension(ex)))
{
GetIconPath = @"C:\Windows\Explorer.exe";
}
if(String.IsNullOrEmpty(sc.Path))
{
sc.Path = @"C:\Windows\Explorer.exe";
GetIconPath = sc.Path;
}
// アイコンの取得
Icon ico = Icon.ExtractAssociatedIcon(GetIconPath);
BitmapSource bs = Imaging.CreateBitmapSourceFromHIcon(ico.Handle, Int32Rect.Empty, itmapSizeOptions.FromEmptyOptions());
sc.Img = bs;
return sc;
}